Memory leaks might sound like something out of a sci-fi movie, but they're actually a common problem in software development. Imagine your computer's memory as a big bucket of water. Now, every time you run an application, it dips into that bucket to borrow some water (memory). But what happens if the app forgets to return that water? Over time, the bucket gets emptier and emptier until your system starts to slow down—or even crashes. That's essentially what a memory leak is, and it's a big deal for developers and users alike.
Now, you might be wondering, "Why should I care about memory leaks?" Well, if you're someone who uses apps, games, or any kind of software, you've probably experienced the frustration of a program suddenly freezing or becoming unresponsive. Often, that's because of a memory leak. And if you're a developer, ignoring memory leaks can lead to angry users, bad reviews, and a tarnished reputation. So, yeah, it's a pretty big deal.
In this article, we're going to break down everything you need to know about memory leaks—what they are, why they happen, and most importantly, how to fix them. Whether you're a coding pro or just someone who wants to understand why their computer keeps slowing down, this guide has got you covered. So buckle up, because we're about to dive deep into the world of memory management!
Read also:Exclusive Leaks Camilla Araujos Story
Here's a quick overview of what we'll cover:
- What is a Memory Leak?
- Causes of Memory Leaks
- Types of Memory Leaks
- Detecting Memory Leaks
- Fixing Memory Leaks
- Memory Leaks in Different Programming Languages
- Tools for Memory Leak Detection
- Real-World Examples of Memory Leaks
- Preventing Memory Leaks
- Conclusion
What is a Memory Leak?
Alright, let's get straight to the point. A memory leak occurs when a program allocates memory but fails to release it back to the system when it's no longer needed. Think of it like borrowing a book from the library but never returning it. Eventually, the library runs out of books, right? Similarly, if too much memory is leaked, your system can run out of resources, leading to crashes or sluggish performance.
Memory leaks are sneaky because they don't always cause immediate problems. At first, you might not even notice them. But over time, as more and more memory is leaked, the effects start to pile up. Your app might slow down, consume excessive memory, or even crash. And if you're running a server or a long-running application, memory leaks can become a nightmare.
Why Should You Care About Memory Leaks?
Here's the deal: memory leaks aren't just annoying—they can be costly. For developers, fixing memory leaks means debugging code, which can take hours or even days. And for users, memory leaks mean slower performance, unexpected crashes, and a frustrating experience. In today's competitive software market, that kind of thing can kill your app's reputation.
So whether you're building the next big app or just using one, understanding memory leaks is crucial. It's like knowing how to change a tire—it might not seem important until you're stranded on the side of the road.
Causes of Memory Leaks
Memory leaks don't just happen out of nowhere. They're usually caused by mistakes in programming or poor memory management practices. Let's break down some of the most common culprits:
Read also:Hot Ullu
- Improper Resource Management: This is like leaving the faucet running after you're done washing your hands. If a program allocates memory but forgets to deallocate it, that memory is essentially lost.
- Circular References: Imagine two objects holding references to each other. Neither can be garbage collected because they're both "in use." This creates a memory leak that's hard to detect.
- Unclosed Connections: If you open a file, database connection, or network socket but forget to close it, the memory associated with that resource remains allocated.
- Global Variables: Global variables can linger around for the entire lifetime of an application, consuming memory unnecessarily.
These are just a few examples, but the root cause of memory leaks often boils down to one thing: forgetting to clean up after yourself. It's like leaving trash on the floor—it might not seem like a big deal at first, but eventually, it becomes a huge mess.
Types of Memory Leaks
Not all memory leaks are created equal. Depending on how they occur, memory leaks can be classified into different types. Here are the main ones:
1. Dangling Pointers
A dangling pointer is like pointing to a house that no longer exists. When a program tries to access memory that has already been freed, it can lead to unpredictable behavior—or worse, a crash.
2. Memory Fragmentation
Memory fragmentation happens when your program allocates and deallocates memory in a way that creates "holes" in the available memory. It's like trying to fit a big puzzle piece into a tiny space—it just doesn't work.
3. Lost References
This happens when a program loses track of allocated memory. It's like forgetting where you parked your car—you know it's somewhere, but you can't find it.
Each type of memory leak has its own quirks and challenges, but the good news is that with the right tools and techniques, you can detect and fix them.
Detecting Memory Leaks
Now that we know what memory leaks are and why they happen, let's talk about how to find them. Detecting memory leaks can be tricky, but there are several methods and tools that can help:
- Manual Code Review: Sometimes, the best way to find memory leaks is to go through your code line by line. Look for places where memory is allocated but not released.
- Profiling Tools: Tools like Valgrind, LeakSanitizer, and Visual Studio's built-in memory profiler can help you identify memory leaks in your code.
- Logging: Adding logging statements to your code can help you track memory usage and identify potential leaks.
Remember, detecting memory leaks is only half the battle. Once you find them, you need to fix them—and that's where things can get tricky.
Fixing Memory Leaks
Fixing memory leaks requires a combination of good coding practices and debugging skills. Here are some tips to help you get started:
- Use Smart Pointers: In languages like C++, smart pointers can help manage memory automatically, reducing the risk of leaks.
- Follow RAII Principles: Resource Acquisition Is Initialization (RAII) is a programming technique that ensures resources are properly managed throughout their lifecycle.
- Test Early and Often: Don't wait until the end of development to test for memory leaks. Test your code regularly to catch issues early.
Fixing memory leaks isn't always easy, but with the right approach, you can prevent them from causing problems down the line.
Memory Leaks in Different Programming Languages
Memory leaks can occur in almost any programming language, but some languages are more prone to them than others. Here's a quick look at how memory leaks manifest in different languages:
1. C and C++
C and C++ are notorious for memory leaks because they give developers full control over memory management. While this can be powerful, it also means you're responsible for cleaning up after yourself.
2. Java and Python
Java and Python have garbage collectors that automatically manage memory, but they're not foolproof. Circular references and improper use of global variables can still lead to memory leaks.
3. JavaScript
JavaScript is another language with a garbage collector, but event listeners and closures can create memory leaks if not handled properly.
Each language has its own quirks when it comes to memory leaks, so it's important to understand how memory management works in the language you're using.
Tools for Memory Leak Detection
There are plenty of tools available to help you detect and fix memory leaks. Here are some of the most popular ones:
- Valgrind: A powerful tool for detecting memory leaks in C and C++ programs.
- LeakSanitizer: Part of the AddressSanitizer suite, LeakSanitizer helps detect memory leaks in C and C++ code.
- Visual Studio Memory Profiler: A built-in tool in Visual Studio that helps you analyze memory usage in your applications.
- Chrome DevTools: For JavaScript developers, Chrome DevTools provides a memory profiler that can help detect memory leaks in web applications.
These tools can save you hours of debugging time, so it's worth investing the time to learn how to use them effectively.
Real-World Examples of Memory Leaks
Memory leaks aren't just a theoretical problem—they happen in real-world applications all the time. Here are a few examples:
- Internet Explorer: Back in the day, Internet Explorer was notorious for memory leaks caused by circular references between JavaScript and the DOM.
- Windows: Some versions of Windows have had memory leak issues, especially in long-running services.
- Games: Memory leaks in video games can cause performance issues, especially in games that run for extended periods.
These examples show that memory leaks can affect even the biggest and most well-known software projects. That's why it's so important to take them seriously.
Preventing Memory Leaks
Preventing memory leaks is all about good coding practices and vigilance. Here are some tips to help you avoid memory leaks in your own projects:
- Write Clean Code: Avoid unnecessary memory allocations and make sure to release resources when they're no longer needed.
- Use Modern Libraries: Libraries and frameworks often have built-in mechanisms to prevent memory leaks, so take advantage of them.
- Test Regularly: Regular testing can help you catch memory leaks early, before they become a bigger problem.
By following these best practices, you can reduce the risk of memory leaks in your applications and ensure a smoother user experience.
Conclusion
Memory leaks might not be the sexiest topic in software development, but they're definitely one of the most important. From causing performance issues to crashing entire systems, memory leaks can have serious consequences for both developers and users. But with the right knowledge, tools, and techniques, you can detect and fix memory leaks before they cause problems.
So the next time you're working on a project, take a moment to think about memory management. Are you cleaning up after yourself? Are you using the right tools to detect potential leaks? These small steps can make a big difference in the long run.
And if you're a user who's experiencing slow performance or crashes, don't just assume it's your computer. It might be a memory leak at work. Share this article with your developer friends, and let's work together to make software better for everyone!
Thanks for reading, and don't forget to leave a comment or share this article if you found it helpful. Until next time, stay leak-free!
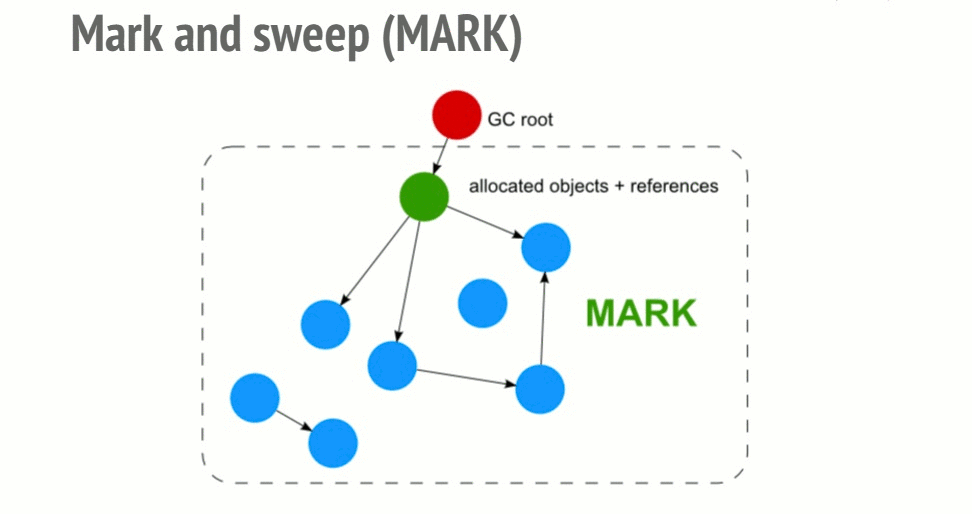

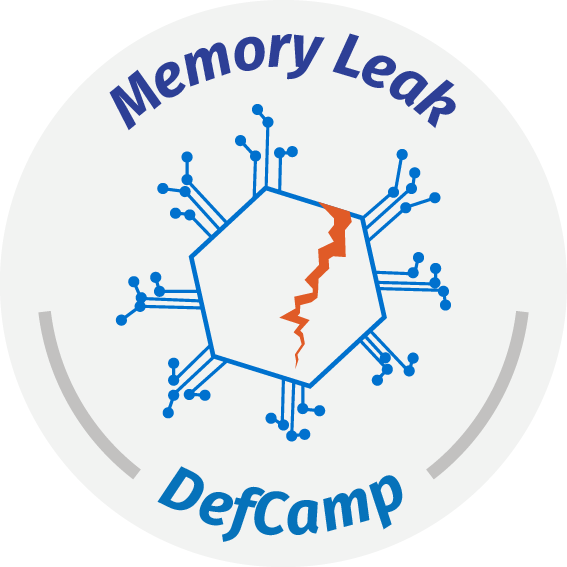